Laravel API development
PHP is among the most widely used web languages today since it is easy to maintain and quick to create a feature-rich Laravel application.
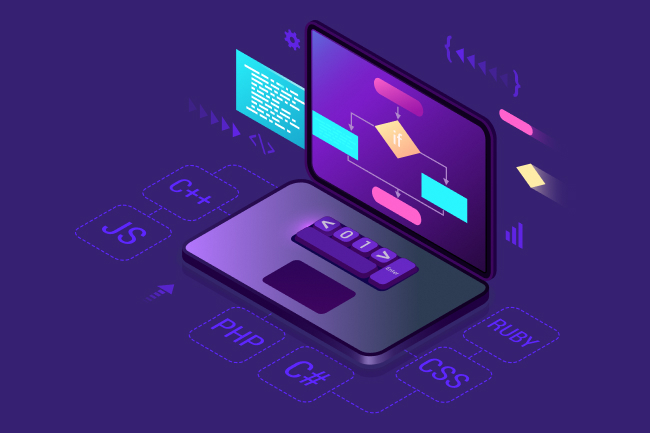
But websites built using Laravel require a powerful tool suite for creating and consuming application programming interfaces (APIs). An appropriate backend API is necessary for developing high-quality content and achieving business goals. Below, we will analyze how to use Laravel API development services to build a PHP RESTful API.
Prerequisites
This guide uses the latest version of the Laravel framework. It also assumes you have a basic knowledge of working on a Laravel project and RESTful APIs. If you are new to Laravel API development, building a Laravel CRUD Application with API Authentication will be a better option for you. Besides, you also need to have the following:
- Composer (a package manager for PHP)
- MySQL
- Postman
- A free Auth0 account
- PHP 7.3 or higher
What are API resources?
API resources allow transforming our models smoothly into JSON responses. That serves as a transformation layer existing between eloquent models and the JSON responses. Also, our Laravel API returns such responses to the API user. API resources consist of two entities called a resource call and a resource collection. While the first corresponds to a single model that must be transformed into a relevant JSON structure, the second is applied to transform a collection of models into this structure. Both can be created using the command line:
// create a resource class
$ php artisan make:resource UserResource
// create a resource collection using either of the two commands
$ php artisan make:resource Users --collection
$ php artisan make:resource UserCollection
What will we build?
We will create a simple Laravel RESTful API using a single /comment resource. This REST (representational state transfer) API will make it possible for everyone to view comments. But at the same time, only authorized users can create, update, and delete comments.
How to set up your Laravel application?
Installation
You need to start by building a new Laravel application using the appropriate PHP service.
Firstly, you must ensure you have already installed a Composer, and then perform the
following command:
composer create-project laravel/laravel laravel-api-auth
cd laravel-api-auth
php artisan serve
Creating comment migration
Now, there is the time for creating the migration. To do that, you should run the following:
php artisan make:migration create_comments_table
That allows creating a new migration file in a relevant directory. The name of the particular file starts with the timestamp of when it was created and then is followed by create_comments_table.php.
After that, you can perform the migration in a terminal using: php artisan migrate
Creating the comment seeder
Building a seeder allows adding mock data to a relevant database table easily using a single command. To create a large API using Laravel, your Laravel web developer may create separate seeder files for all models by performing php artisan make:seeder CommentSeeder.
Then you need to open up /database/seeders/DatabaseSeeder.php and update it in the
next way:
{{"
namespace Database\Seeders;
use Illuminate\Database\Seeder;
use App\Models\Comment;
class DatabaseSeeder extends Seeder
{
/**
* Seed the application's database.
*
* @return void
*/
public function run()
{
Comment::factory()
->times(3)
->create();
}
}
Creating comment factory
You should create your comment factory by performing the following command:
php artisan make:factory CommentFactory
Then, you must open up the newly created file existing in
database/factories/CommentFactory.php and replace it with such a command:
{{"
namespace Database\Factories;
use App\Models\Comment;
use Illuminate\Database\Eloquent\Factories\Factory;
use Illuminate\Support\Str;
class CommentFactory extends Factory
{
/**
* The name of the factory's corresponding model.
*
* @var string
*/
protected $model = Comment::class;
/**
* Define the model's default state.
*
* @return array
*/
public function definition()
{
return [
'name' =>
$this->faker->name,
'text' =>
$this->faker->text()
];
}
}
Creating the comment controller
To create the comment controller, you should perform the next command in your terminal:
php artisan make:controller API/CommentController --resource
That allows creating a new file at app/Http/Controllers/API/CommentController.php.
Then, you
should open this file and update it using this command line:
{{"
use App\Http\Controllers\Controller;
use App\Models\Comment;
use Illuminate\Http\Request;
class APIController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$comments = Comment::all();
return response()->json($comments);
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'name' =>
'required|max:255',
'text' =>
'required'
]);
$newComment = new Comment([
'name' =>
$request->get('name'),
'text' =>
$request->get('text')
]);
$newComment->save();
return
response()->json($newComment);
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
$comment =
Comment::findOrFail($id);
return response()->json($comment);
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
$comment =
Comment::findOrFail($id);
$request->validate([
'name' =>
'required|max:255',
'text' =>
'required'
]);
$comment->name =
$request->get('name');
$comment->text =
$request->get('text');
$comment->save();
return response()->json($comment);
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
$comment =
Comment::findOrFail($id);
$comment->delete();
return
response()->json($comment::all());
}
}
Creating API routes
Ultimately, let’s establish the API routes. You should open up routes/api.php for
replacing
everything by running:
{{"
use Illuminate\Http\Request;
Applying Route::apiResource() allows building all of the routes necessary for
creating,
showing, updating, and deleting comments. Also, you can see the particular routes in your
app by running:php artisan route:list
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\API\CommentController;
/*
|-------------------------------------------------------------------
| API Routes
|-------------------------------------------------------------------
|
| Here is where you can register API routes for your app. Such
| routes are loaded by the RouteServiceProvider within a group which
| is assigned the "api" middleware group. Enjoy building your API!
|
*/
Route::apiResource('comments', CommentController::class);
Testing your Laravel API
You need to confirm that your Laravel API development solution performs correctly by
applying Laravel API testing from your terminal using cURL. For example, to get all
necessary comments, you should implement the following cURL command PHP artisan:
curl http://localhost:8000/api/comments -i
Thus, Laravel REST API testing is a critical tool to ensure the appropriate performance of
your Laravel API.
Secure your Laravel API solution
Now, you have a fully functional Laravel API development solution, but you cannot celebrate yet. One of the major constraints of your app is that several API endpoints must be private. However, right now, everyone can conduct different operations on a relevant API. So there is a need to fix that.
Configure Auth0
You should use Auth0 for protecting the private endpoints. If you still have not done that already, you should sign up using a free account. After accessing your dashboard, you must register your Laravel API using Auth0.
Install dependencies
After setting up your API, you need to return to your terminal and run the following
command:
composer require auth0/login
That allows you to install a Laravel plugin called the Auth0 one.
After that, you should generate the specific configuration file. Thus, you have to run:
php artisan vendor:publish --provider
"Auth0\Login\LoginServiceProvider"
Test your protected routes
Here, you should test protected routes one more time to ensure that anyone cannot create,
update, and destroy comments without a relevant access token. But firstly, you need to try
to make new comments using your command line without any tokens:
curl -X POST -H 'Content-Type: application/json' -d '{
That allows returning unauthorized HTTP status codes.
"name": "Lucy",
"text": "An authorized comment"
}' http://localhost:8000/api/comments -i
Conclusion
With Laravel API development, you can build powerful and secure Laravel RESTful API quickly. Using this amazing web application framework allows securing user data, enhancing the source code, providing authorization services, and simplifying the entire route path. It automatically provides the application with all known routes. Ultimately, the Laravel API development solution provides necessary exceptions for application configuration and error handling. That allows tracing and resolving any bugs.
We hope that this tutorial will help you learn how to build the Laravel API appropriately using different methods and authentication. In Flexi IT, we can provide you with high-quality Laravel API development solutions at affordable prices. If you consider integrating APIs into your Laravel applications, please contact us at partners@flexi.ink.
Testimonials
I feel blessed to have discovered Flexi! I was looking for developers who take pride in getting things done, not trying to explain why some of my design ideas might not work. And I found them! I hired Flexi to develop a custom WordPress site that I designed. The final product looked and worked exactly the way I anticipated, and it was delivered on time. Vlad has ...Read more
I've been working with Flexi on my project for a long time. They are always on time. Very loyal service. Hope we'll continue to collaborate for years to come.
Contact us
Try us for 14 days
Want to start a 2-week free trial period with us? Leave your email below and we'll revert to you shortly with more details